Configuring Maven to run JUnit5 unit and integration tests
Table of Contents
In this post I will show how to set up Maven to run unit tests and integration tests correctly. This seemingly simple task requires some effort, but once done correctly you can sleep well and be sure that no one will complain about it. To show the step-by-step process I’ve created simple Maven project.
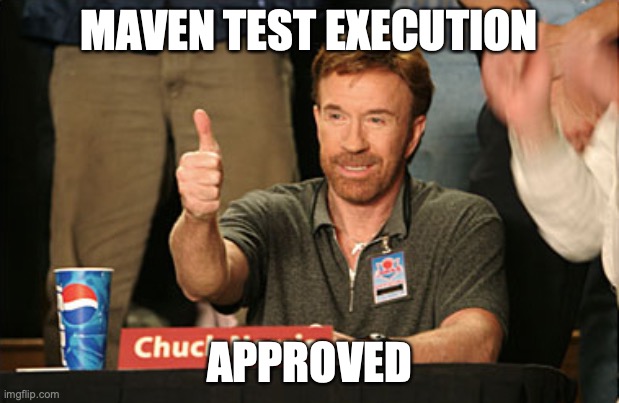
If you have to configure test execution do it right! :)
Example project
Our project structure looks like this:
> tree
.
├── pom.xml - Maven config for our project
└── src
├── main
└── test
└── java
└── codes
└── hubertwo
└── maven
└── tests
├── DummyTest.java - contains unit tests
└── DummyTestIT.java - contains integration tests
The project contains single pom.xml
file with JUnit5 dependency and no additional configuration.
We also some dummy tests under src/test/java
.
Know your toolbox - Maven plugins for running tests
Maven comes with two plugins Surefire and Failsafe to run tests.
The Failsafe Plugin is designed to run integration tests while the Surefire Plugin is designed to run unit tests. The name (failsafe) was chosen both because it is a synonym of surefire and because it implies that when it fails, it does so in a safe way.
From: https://maven.apache.org/surefire/maven-failsafe-plugin/
Executing Maven test goal without configured plugins
If we execute mvn test
without any Maven plugins defined no tests will be executed.
> mvn test
[INFO] Scanning for projects...
...
[INFO] --- maven-surefire-plugin:2.12.4:test (default-test) @ runtests ---
-------------------------------------------------------
T E S T S
-------------------------------------------------------
Results :
Tests run: 0, Failures: 0, Errors: 0, Skipped: 0
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
You may ask: Why Maven doesn’t execute my Junit5 tests?!
The answer is simple maven-surefire-plugin
version we use does not support JUnit5.
Unit tests - Maven Surefire plugin
To simply check which version you use for running test execute command below:
> mvn -Dplugin=org.apache.maven.plugins:maven-surefire-plugin help:describe | grep 'Version:'
Version: 2.12.4
The minimum version of Surefire plugin for JUnit5 is 2.22.0.
Let’s add Surefire plugin with proper version to our pom.xml
file.
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-surefire-plugin</artifactId>
<version>3.0.0-M5</version>
</plugin>
</plugins>
</build>
And now run mvn test
one more time.
% mvn test
[INFO] Scanning for projects...
...
[INFO] --- maven-surefire-plugin:3.0.0-M5:test (default-test) @ runtests ---
[INFO]
[INFO] -------------------------------------------------------
[INFO] T E S T S
[INFO] -------------------------------------------------------
[INFO] Running codes.hubertwo.maven.tests.DummyTest
[INFO] Tests run: 2, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.044 s - in codes.hubertwo.maven.tests.DummyTest
[INFO]
[INFO] Results:
[INFO]
[INFO] Tests run: 2, Failures: 0, Errors: 0, Skipped: 0
[INFO]
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
[INFO] ------------------------------------------------------------------------
Great! Unit tests are picked up by Maven and executed as expected, but what about integration tests? Why they are not executed?
Integration tests - Failsafe plugin
Maven provides dedicated goal for integration tests called integration-test
.
Let’s execute the goal and check the results.
> mvn integration-test
[INFO] --- maven-surefire-plugin:3.0.0-M5:test (default-test) @ runtests ---
[INFO]
[INFO] -------------------------------------------------------
[INFO] T E S T S
[INFO] -------------------------------------------------------
[INFO] Running codes.hubertwo.maven.tests.DummyTest
[INFO] Tests run: 2, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.045 s - in codes.hubertwo.maven.tests.DummyTest
[INFO]
[INFO] Results:
[INFO]
[INFO] Tests run: 2, Failures: 0, Errors: 0, Skipped: 0
Again, no integration tests were executed. This happens because there’s no plugin defined to run integration tests.
It’s time to edit pom.xml
file again and add Failsafe plugin configuration.
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-failsafe-plugin</artifactId>
<version>3.0.0-M1</version>
<executions>
<execution>
<goals>
<goal>integration-test</goal>
<goal>verify</goal>
</goals>
</execution>
</executions>
</plugin>
The goals
section tells Maven when to use plugin.
Looks like we are set and ready to go.
mvn integration-test
[INFO] Scanning for projects...
...
[INFO] --- maven-failsafe-plugin:3.0.0-M1:integration-test (default) @ runtests ---
[INFO]
[INFO] -------------------------------------------------------
[INFO] T E S T S
[INFO] -------------------------------------------------------
[INFO] Running codes.hubertwo.maven.tests.DummyTestIT
[INFO] Tests run: 2, Failures: 0, Errors: 0, Skipped: 0, Time elapsed: 0.028 s - in codes.hubertwo.maven.tests.DummyTestIT
[INFO]
[INFO] Results:
[INFO]
[INFO] Tests run: 2, Failures: 0, Errors: 0, Skipped: 0
[INFO]
[INFO] ------------------------------------------------------------------------
[INFO] BUILD SUCCESS
Awesome! Integration tests are executed as expected!
Summary
In this post we got familiar with Surefire and Failsafe plugins, saw how to configure Maven to find and execute both unit and integration test and troubleshoot the problem with JUnit5 tests.
Source code
All code samples and Maven project described in this post is available on GitHub
Maven unit and integration tests.
If you found this post useful do not forget to leave a ⭐️ on GitHub :) Thanks!
Read more
- Maven build lifecycle Maven goals and execution order explained
- Maven Surefire plugin for unit tests
- Maven Failsafe plugin for integration tests